- November 29, 2024
- Web Development
React JS With Mongodb
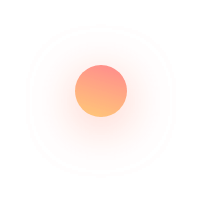
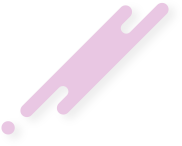
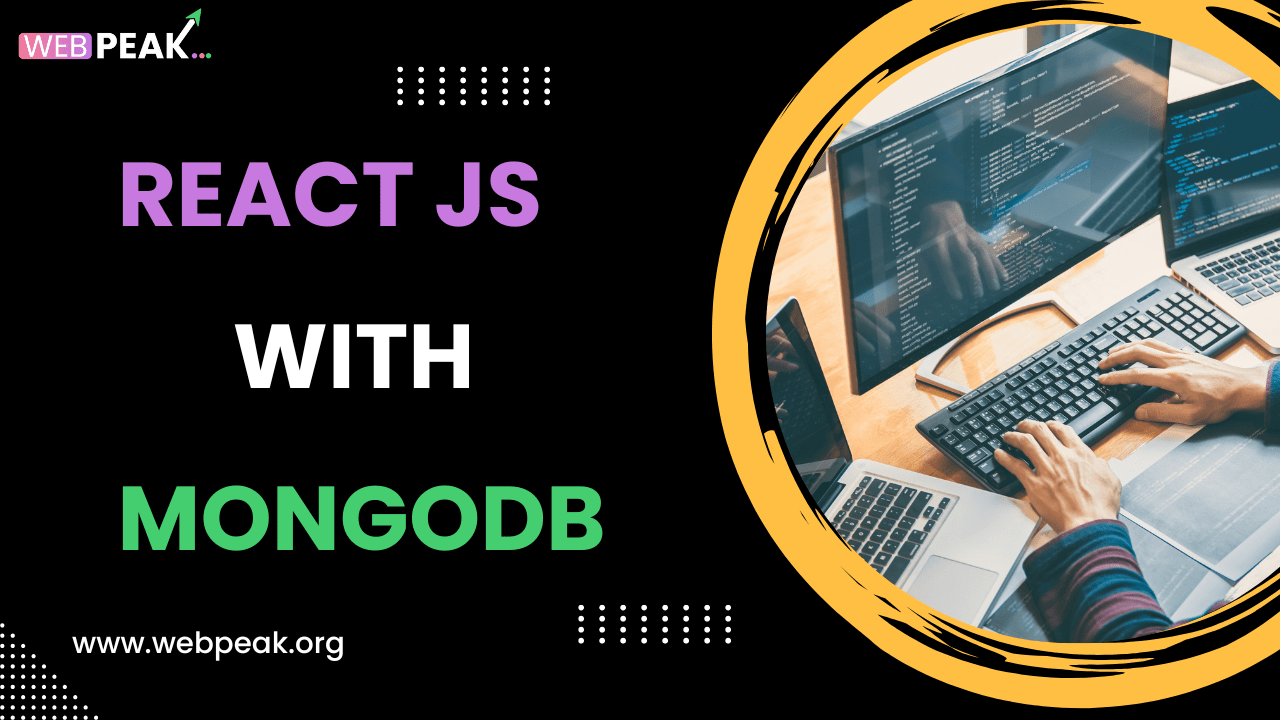
React.js With MongoDB: Building Scalable and Dynamic Applications
The combination of React.js and MongoDB is a popular choice for developers seeking to build scalable, responsive, and modern web applications. React.js, a JavaScript library for building user interfaces, works seamlessly with MongoDB, a NoSQL database known for its flexibility and performance. Together, they empower developers to create full-stack applications that handle data efficiently while offering a rich user experience.
This article explores how React.js and MongoDB integrate to build robust applications, covering their individual strengths, integration methods, and use cases.
What is React.js?
React.js is an open-source JavaScript library developed by Facebook. It is widely used for building dynamic and responsive user interfaces. React.js leverages a component-based architecture, allowing developers to break down complex UIs into reusable components.
Key Features of React.js:
- Virtual DOM: Enables fast updates by rendering only the components that change.
- Component-Based Architecture: Promotes reusability and modularity in code.
- One-Way Data Binding: Ensures predictable and bug-free data flow.
- Rich Ecosystem: Supported by libraries like Redux, React Router, and Material-UI for enhanced functionality.
What is MongoDB?
MongoDB is a NoSQL database designed for handling unstructured and semi-structured data. Unlike traditional relational databases, MongoDB stores data in JSON-like documents, making it a natural fit for JavaScript-based technologies like React.js.
Key Features of MongoDB:
- Flexible Schema: Adapts to changing application needs without requiring migrations.
- Scalability: Designed to handle large volumes of data and horizontal scaling.
- High Performance: Optimized for read and write operations.
- Rich Query Language: Offers powerful querying, indexing, and aggregation capabilities.
Why Combine React.js and MongoDB?
Pairing React.js and MongoDB creates a powerful full-stack solution, particularly when combined with backend technologies like Node.js or Express.js. Here is why they work well together:
- Seamless Data Handling: MongoDB's JSON-like document structure complements React.js’s JavaScript-centric environment.
- Real-Time Updates: Both technologies support real-time operations, enhancing user experience in dynamic applications.
- Scalability: MongoDB’s horizontal scaling capabilities align well with React.js’s ability to manage large-scale user interfaces.
- Developer Productivity: Both technologies are developer-friendly, allowing quick prototyping and deployment.
Setting Up a React.js and MongoDB Project
Step 1: Prerequisites
Before starting, ensure you have the following installed:
- Node.js and NPM
- MongoDB
- A code editor (e.g., VS Code)
Step 2: Setting Up MongoDB
- Install MongoDB and start the database service.
- Use the MongoDB shell or MongoDB Compass to create a new database, for example,
myAppDB
. - Create a collection, such as
users
, to store sample data.
Step 3: Setting Up the Backend with Node.js and Express
Create a backend API using Node.js and Express to interact with MongoDB. This API will serve as a bridge between React.js and MongoDB.
Backend Structure:
/backend /models User.js /routes userRoutes.js server.js
Example Backend Code:
server.js:
const express = require('express'); const mongoose = require('mongoose'); const userRoutes = require('./routes/userRoutes'); const app = express(); app.use(express.json()); app.use('/api/users', userRoutes); mongoose.connect('mongodb://localhost:27017/mapped', { useNewUrlParser: true, useUnifiedTopology: true, }).then(() => console.log('Connected to MongoDB')) .catch(err => console.log(err)); app.listen(5000, () => console.log('Server running on port 5000'));
Step 4: Creating the React Frontend
Set up the React application using the create-react-app command:
npx create-react-app frontend cd frontend
Fetching Data from the Backend:
import React, { useEffect, useState } from 'react'; import axios from 'axios'; function App() { const [users, setUsers] = useState([]); useEffect(() => { axios.get('http://localhost:5000/api/users') .then(response => setUsers(response.data)) .catch(error => console.error('Error fetching users:', error)); }, []); return ( <div> <h1>User List</h1> {users.map(user => ( <p key={user._id}>{user.name} ({user.email})</p> ))} </div> ); } export default App;
Best Practices for React.js and MongoDB Integration
- Use Environment Variables to store sensitive information, such as database URIs, in
.env
files. - Implement Authentication in your application using tools like JWT or OAuth.
- Paginate Data: Use MongoDB’s
limit
andskip
queries to handle large datasets efficiently. - Optimize API calls by caching data in the frontend using libraries like React Query.
- Error Handling: Implement robust error-handling mechanisms on both the frontend and backend.
Popular Use Cases
- E-Commerce Applications: React.js handles dynamic product listings, while MongoDB stores product and user data.
- Social Media Platforms: MongoDB’s flexibility is ideal for managing varied content types like posts, comments, and media.
- Real-Time Chat Applications: React.js and MongoDB (with WebSocket support) enable seamless messaging experiences.
- Content Management Systems: MongoDB’s schema-less structure adapts to diverse content types, and React.js provides a user-friendly UI.
Conclusion
Combining React.js and MongoDB allows developers to build modern, scalable, and feature-rich web applications. React.js excels at creating interactive user interfaces, while MongoDB efficiently handles data storage and retrieval. Together, they provide the tools needed to tackle a wide range of real-world use cases.
By following best practices and leveraging the strengths of both technologies, developers can create robust applications that delight users and meet business goals. Whether you are building a small project or a large-scale application, React.js with MongoDB is a powerful and flexible choice.
WebPeak Blog
Best Web Development Company
By Web DevelopmentWebPeak.org is a top web development company offering custom, SEO-friendly, and scalable websites with expert support and transparent pricing.
Read MoreLocal SEO Croydon
By Digital MarketingMaster local SEO for your Croydon business. Drive foot traffic, boost Google rankings, and grow your brand with proven local strategies.
Read MoreTop Reasons Why Businesses Should Opt for WordPress Development
By Web DevelopmentChoose WordPress for your business—easy to use, SEO-friendly, customizable, and backed by expert developers for top-tier results.
Read More